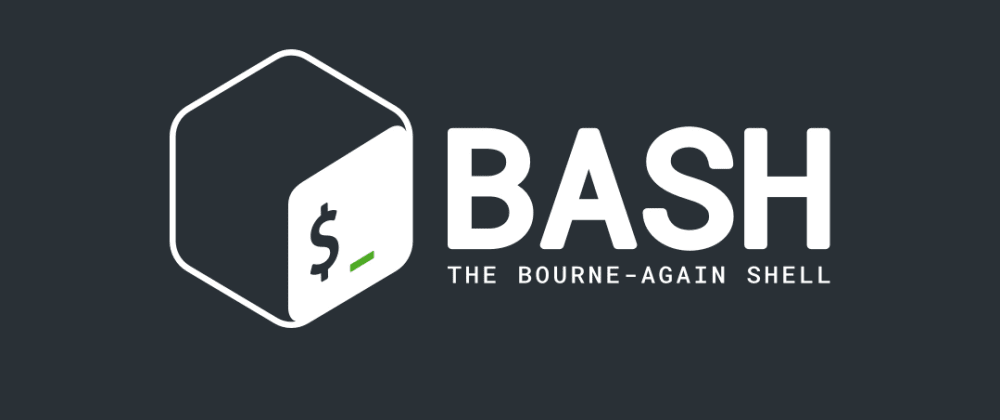
Hey guys, Rocky here! đ
Today, weâre diving into Bash Scriptingâthe backbone of automation and system management in Linux/Unix environments. Whether youâre a developer, sysadmin, or just a tech enthusiast, mastering Bash scripting will save you time, reduce repetitive tasks, and unlock the true power of the command line. Letâs break it down step by step!
â
What is Bash?
Bash (Bourne Again SHell) is a command-line interpreter and scripting language. Itâs the default shell on most Linux distributions and macOS (though macOS is moving to Zsh). Bash allows you to execute commands, automate workflows, and even build complex programs using simple scripts.
â
Why Learn Bash Scripting?
- Automate the boring stuff: Schedule backups, clean up files, or deploy code.
- System management: Monitor resources, manage users, or configure servers.
- Portability: Bash scripts run on almost any Unix-like system.
- Foundation for DevOps: Tools like Docker, Kubernetes, and CI/CD pipelines rely on shell scripting.
â
Getting Started: Your First Script
Letâs create a simple âHello Worldâ script.
Create a file:
Open a terminal and type:
nano hello.sh
Add the shebang line:
The #!/bin/bash
(shebang) tells the system to use Bash to execute the script.
#!/bin/bash
echo "Hello World!"
Make it executable:
chmod +x hello.sh
Run the script:
./hello.sh # Output: Hello World!
â
Bash Scripting Basics
1. Variables
Variables store data. No data typesâeverything is a string (unless you enforce arithmetic).
name="Rocky"
age=30
echo "Hey, I'm $name and Iâm $age years old."
- Note: No spaces around
=
in assignments!
2. Comments
Use #
for single-line comments:
# This is a comment
3. Input/Output
Printing: echo "Text"
or printf "Formatted Text"
Reading input:
echo "What's your name?"
read username
echo "Hello, $username!"
â
Control Structures
1. Conditionals (if/else)
Check conditions using if
, elif
, and else
:
if [ $age -gt 18 ]; then
echo "Youâre an adult."
elif [ $age -eq 18 ]; then
echo "Welcome to adulthood!"
else
echo "Youâre a minor."
fi
Comparison Operators:
-eq
: Equal
-ne
: Not equal
-gt
: Greater than
-lt
: Less than
-z "string"
: Check if string is empty
2. Case Statements
Simplify multiple conditions:
case $day in
"Mon") echo "Monday Blues!" ;;
"Fri") echo "TGIF!" ;;
*) echo "Itâs $day. Keep going!" ;;
esac
3. Loops
For Loop:
for i in {1..5}; do
echo "Count: $i"
done
While Loop:
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
((count++))
done
Until Loop:
Runs until a condition becomes true.
until [ $count -gt 5 ]; do
echo "Count: $count"
((count++))
done
â
Functions
Reuse code with functions:
greet() {
echo "Hello, $1!" # $1 = first argument
}
greet "Rocky" # Output: Hello, Rocky!
- Return values: Use
return
for numeric status (0 = success, non-zero = error).
â
Handling Arguments
Scripts accept command-line arguments:
$0
: Script name
$1
, $2
, âŠ: Arguments
$#
: Number of arguments
$@
: All arguments as a list
Example:
#!/bin/bash
echo "Script: $0"
echo "First arg: $1"
echo "All args: $@"
â
Exit Codes & Error Handling
Every command returns an exit code (0
= success, 1-255
= error).
Use exit
to set a scriptâs exit code:
if [ ! -f "file.txt" ]; then
echo "Error: File not found!" >&2 # Redirect to stderr
exit 1
fi
â
Debugging Bash Scripts
Enable debugging:
bash -x script.sh # Prints each command before execution
Add set -e
: Exit immediately if any command fails.
Add set -o pipefail
: Catch errors in pipelines.
â
Best Practices
Quote variables: Prevent word splitting.
echo "$name" # Safer than echo $name
Use [[ ]]
instead of [ ]
: More features and fewer surprises.
Check for dependencies:
if ! command -v git &> /dev/null; then
echo "Install git first!"
exit 1
fi
Indent code: Improve readability.
â
Example Script: Backup Utility
Putting it all together:
#!/bin/bash
# Backup files in a directory
backup_dir="/home/rocky/backups"
source_dir="/home/rocky/documents"
if [ ! -d "$source_dir" ]; then
echo "Error: Source directory missing!" >&2
exit 1
fi
mkdir -p "$backup_dir"
tar -czf "$backup_dir/backup_$(date +%Y%m%d).tar.gz" "$source_dir"
echo "Backup completed!"
â
Next Steps
- Practice writing scripts for daily tasks.
- Explore advanced topics: arrays, traps, subshells, and regex.
- Read the Bash Manual.
Remember: The best way to learn is by breaking things and fixing them. đ
Ready to Level Up Your Bash Skills? đ
If youâre serious about mastering Bash scripting and want to dive deeper into building custom tools, automating complex workflows, or even integrating scripting into penetration testing (yes, weâre talking ethical hacking here!), check out our comprehensive guide: Master Shell Scripting: Build Custom Tools & Automate Pentesting. This book is packed with hands-on projects, real-world examples, and advanced techniques to transform you from a Bash novice to a scripting wizard. Whether youâre automating server deployments, parsing logs for security analysis, or crafting your own CLI utilities, this guide has you covered. Grab your copy today and start turning those repetitive tasks into one-click solutions! đ„
â
Rocky out! đ