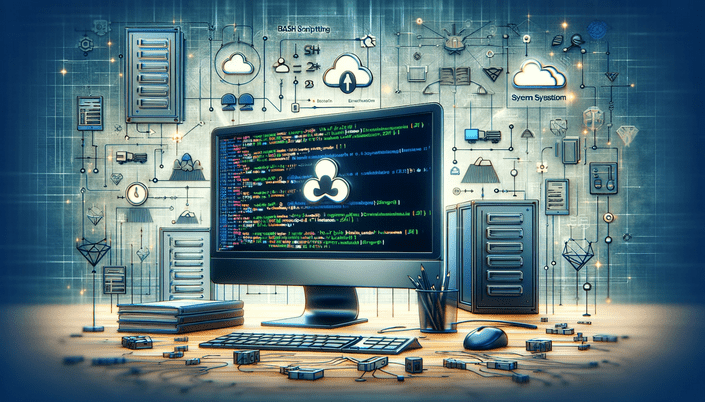
One of the most powerful skills you can acquire as a novice DevOps engineer is Bash scripting. Using Bash scripts, you can automate your processes and save time, reduce human mistakes, and become more efficient. You can use these basic Bash scripts for server management, monitoring, backups, or deployments. Below are 10 essential Bash scripts for novice DevOps engineers.
โ
1๏ธโฃ Automate System Updates ๐
This script ensures your system is always up-to-date with the latest patches and security updates.
\#!/bin/bash
# Update system packages
sudo apt update && sudo apt upgrade -y
sudo apt autoremove -y
sudo apt clean
echo "System updated successfully!"
๐น How it helps: Automates routine system maintenance to keep your environment secure and up-to-date.
โ
2๏ธโฃ Backup Files or Directories ๐๏ธ
Regular backups are critical in any DevOps environment. Use this script to backup files or directories.
#!/bin/bash
# Backup important files or directories
SOURCE="/path/to/source"
DESTINATION="/path/to/backup"
DATE=$(date +%F)
tar -czvf $DESTINATION/backup_$DATE.tar.gz $SOURCE
echo "Backup completed successfully!"
๐น How it helps: Automates backups and keeps versioned copies of your important files.
โ
3๏ธโฃ Monitor Disk Usage ๐
Keep track of your systemโs disk usage and alert you when space is low.
#!/bin/bash
# Check disk usage and send alert if it's more than 90%
USAGE=$(df -h | grep '/dev/sda1' | awk '{ print $5 }' | sed 's/%//g')
if [ $USAGE -gt 90 ]; then
echo "Warning: Disk space is critically low at $USAGE%" | mail -s "Disk Space Alert" admin@example.com
fi
๐น How it helps: Monitors disk usage and sends an alert when space is low, helping you prevent server issues.
โ
4๏ธโฃ Automate Log Rotation ๐
Log files can grow quickly. This script automatically rotates logs to manage log file sizes.
#!/bin/bash
# Rotate logs to prevent large log file sizes
LOG_DIR="/var/log"
LOG_FILE="application.log"
ARCHIVE_DIR="/path/to/archive"
DATE=$(date +%F)
mv $LOG_DIR/$LOG_FILE $ARCHIVE_DIR/application_$DATE.log
touch $LOG_DIR/$LOG_FILE
echo "Log rotation completed successfully!"
๐น How it helps: Prevents your logs from becoming too large and causing storage issues.
โ
5๏ธโฃ Create a New User and Add to Group ๐ฅ
This script automates the process of adding a new user and assigning them to a specific group.
#!/bin/bash
# Create a new user and add to a group
USERNAME=$1
GROUPNAME=$2
# Create user and assign to group
sudo useradd -m -g $GROUPNAME $USERNAME
echo "User $USERNAME created and added to $GROUPNAME successfully!"
๐น How it helps: Quickly creates users and organizes them into the appropriate groups for better access management.
โ
6๏ธโฃ Clean Up Old Files ๐งน
Automatically delete old files that are no longer needed, such as temporary files or logs.
#!/bin/bash
# Remove files older than 30 days
find /path/to/directory -type f -mtime +30 -exec rm -f {} \;
echo "Old files removed successfully!"
๐น How it helps: Prevents disk space issues by cleaning up outdated files automatically.
โ
7๏ธโฃ Automate Service Restart ๐
This script allows you to automatically restart a service, for example, when an update is applied or the service is down.
#!/bin/bash
# Restart a service
SERVICE=$1
sudo systemctl restart $SERVICE
echo "$SERVICE restarted successfully!"
๐น How it helps: Ensures services are always running and up-to-date with minimal manual intervention.
โ
8๏ธโฃ Check Service Status โ๏ธ
This script checks whether a particular service is running and sends an alert if itโs not.
#!/bin/bash
# Check if a service is running
SERVICE=$1
if systemctl is-active --quiet $SERVICE; then
echo "$SERVICE is running."
else
echo "$SERVICE is not running. Sending alert..."
mail -s "$SERVICE down" admin@example.com
fi
๐น How it helps: Automates the monitoring of critical services, sending alerts when thereโs an issue.
โ
9๏ธโฃ Automate Deployment ๐
This script automates the deployment process for your applications, making it easier to deploy code with minimal steps.
#!/bin/bash
# Deploy application by pulling the latest code from Git and restarting the service
cd /path/to/app
git pull origin main
sudo systemctl restart app.service
echo "Deployment completed successfully!"
๐น How it helps: Streamlines the deployment process and minimizes human errors during code updates.
โ
๐ Set Up Cron Jobs ๐
Set up cron jobs to automate tasks at specific intervals. For example, run a cleanup script every Sunday.
#!/bin/bash
# Set up cron job to run every Sunday at midnight
(crontab -l 2>/dev/null; echo "0 0 * * SUN /path/to/cleanup.sh") | crontab -
echo "Cron job set successfully!"
๐น How it helps: Automates repetitive tasks by scheduling them at specific times, saving you manual effort.
โ
Bonus: Master Bash & Python for Cyber Exploits and Automation ๐
To take your Bash and automation skills even further, check out our book:
In this book, youโll learn how to use Python and Bash to automate cybersecurity tasks, craft tools for penetration testing, and handle automation within a red team environment. Whether youโre looking to improve your cyber exploitation skills or need to automate common cybersecurity workflows, this book is packed with practical examples and strategies.
โ
Conclusion: Why Bash Scripting is Key to DevOps ๐
Bash scripting is one of the most valuable tools for any DevOps engineer. By automating common tasks like backups, system updates, service monitoring, and deployments, you can save time, reduce errors, and improve efficiency. The scripts listed above are just the beginningโonce you get the hang of Bash, youโll be able to automate almost any task in your DevOps workflow!
๐ฌ Which Bash script will you automate first? Let me know in the comments below! ๐
โ